Web3.js HTML Example: A Guide to Using Web3.js in Your Next Project
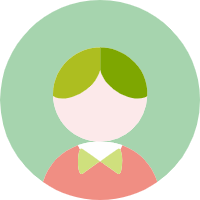
Web3.js is a popular library that enables web developers to interact with blockchain technology, such as Ethereum and Bitcoin. It provides a simple and reliable way to send transactions, connect to a node, and manage contracts on the blockchain. In this article, we will explore a simple HTML example that demonstrates how to use Web3.js in your next project.
Step 1: Install Web3.js
First, you need to install Web3.js on your development environment. You can do this using npm (Node.js package manager) or yarn. Open a terminal or command prompt and run the following command:
```
npm install web3
```
Or, if you're using yarn:
```
yarn add web3
```
Step 2: Set up a simple transaction
Web3.js provides a simple way to send transactions to the blockchain. Let's create a transaction that sends 0.5 ether (ETH) to another address. Create a new HTML file and add the following code:
```html
Web3.js HTML Example
Simple Transaction Example
Send 0.5 ETH
```
Step 3: Create a JavaScript file and setup Web3.js
Create a new JavaScript file (e.g., script.js) and add the following code:
```javascript
const Web3 = require('web3');
const web3 = new Web3('https://mainnet.infura.io/v3/YOUR_INFURA_PROJECT_ID');
async function sendTransaction() {
const nonce = await web3.eth.getTransactionCount('your_address_here');
const txData = '0x' + web3.eth.accounts.encryptTransactionData({ from: 'your_address_here', to: 'the_recipient_address', value: 0.5 });
const tx = { data: txData, nonce: parseInt(nonce) };
try {
const sent = await web3.eth.sendSignedTransaction(tx);
console.log(`Transaction sent: ${sent.transactionHash}`);
document.getElementById('result').innerHTML = `Transaction sent successfully!`;
} catch (error) {
console.error(`Error sending transaction: ${error.message}`);
document.getElementById('result').innerHTML = `Error sending transaction.`;
}
}
```
Step 4: Connect to a node
Web3.js also enables you to connect to a node on the blockchain. You can use Infura (https://infura.io/) to connect to a node for free. Replace 'YOUR_INFURA_PROJECT_ID' with your Infura project ID in the code above.
Step 5: Run the Application
Now, you can run your application in a browser. Open the HTML file in a web browser and click the "Send 0.5 ETH" button. You should see a message indicating whether the transaction was sent successfully.
Web3.js is a powerful library that makes it easy to integrate blockchain technology into your web applications. By following this guide, you've seen a simple example of using Web3.js to send transactions and connect to a node. Feel free to explore more advanced features of Web3.js, such as managing smart contracts, as you develop your next project.